MFMailComposeViewController - sending emails from an iOS app
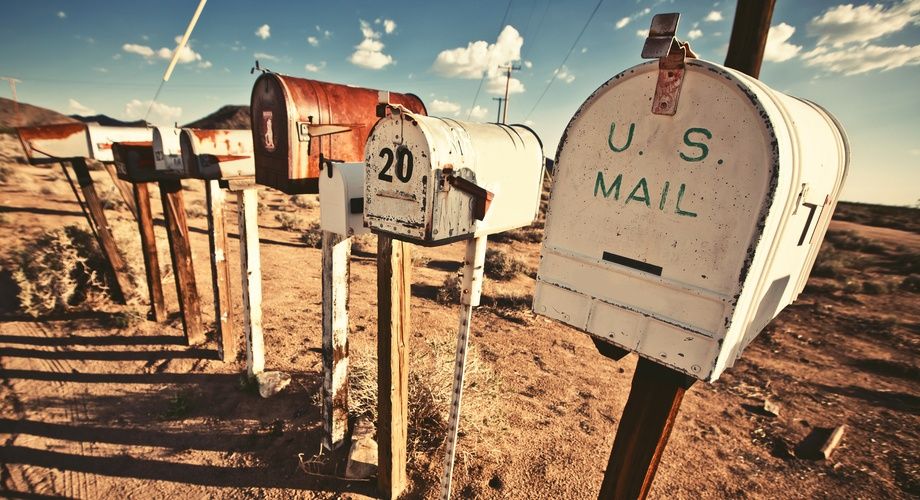
MFMailComposeViewController provides an easy way to present a view controller, that allows to write, edit and send an email. It’s presented modally, so the context of the app won’t be left.
Hint: This post is using Swift 4 and iOS 9
Creating and presenting MFMailComposeViewController
It’s very easy. First, you have to import the MessageUI framework:
import MessageUI
Then, you just have to create an instance of MFMailComposeViewController, set some parameters and present it. The rest is taken care of by that controller.
if MFMailComposeViewController.canSendMail() { let mailComposeViewController = MFMailComposeViewController() mailComposeViewController.mailComposeDelegate = self mailComposeViewController.setToRecipients(["abc@abc.abc"]) mailComposeViewController.setSubject("Subject") mailComposeViewController.setMessageBody("Hello!!!", isHTML: false) present(mailComposeViewController, animated: true, completion: nil) }
The static canSendMail() method of MFMailComposeViewController is called to check if an email account is set up on the device. Note that this doesn’t include email accounts of third party apps like Gmail or Outlook.
It’s important to set the MFMailComposeViewControllerDelegate as well to handle the dismissal of the controller. There’s just one method to implement:
func mailComposeController(_ controller: MFMailComposeViewController, didFinishWith result: MFMailComposeResult, error: Error?) { controller.dismiss(animated: true, completion: nil) }
It’s called when the user dismisses the controller, either by sending the email or canceling the action. Either way, you have to dismiss the controller manually.
Setting the recipients, subject and message body are the most obvious parameters you can set. However, it’s also possible to set CC and BCC recipients:
mailComposeViewController.setCcRecipients(["cc@cc.cc"]) mailComposeViewController.setBccRecipients(["bcc@bcc.bcc"])
It’s also possible to set an attachment:
if let image = UIImage(named: "Test"), let imageData = UIImageJPEGRepresentation(image, 1.0) { mailComposeViewController.addAttachmentData(imageData, mimeType: "image/jpeg", fileName: "Image.jpeg") }
In this example, an image called Test.jpeg from the asset bundle gets attached to the email.
Open Mail app
There’s also another way to sent emails from an iOS app. Instead of presenting the MFMailComposeViewController, you can open the mail app:
if let url = URL(string: "mailto:abc@abc.abc") { UIApplication.shared.open(url, options: [:], completionHandler: nil) }
There is also the possibility to use the UIActivityViewController. However, then the user can choose from different types of channels, not only email.
References
Title image: @ Andrey Bayda / shutterstock.com