Swift: The Nil Coalescing Operator
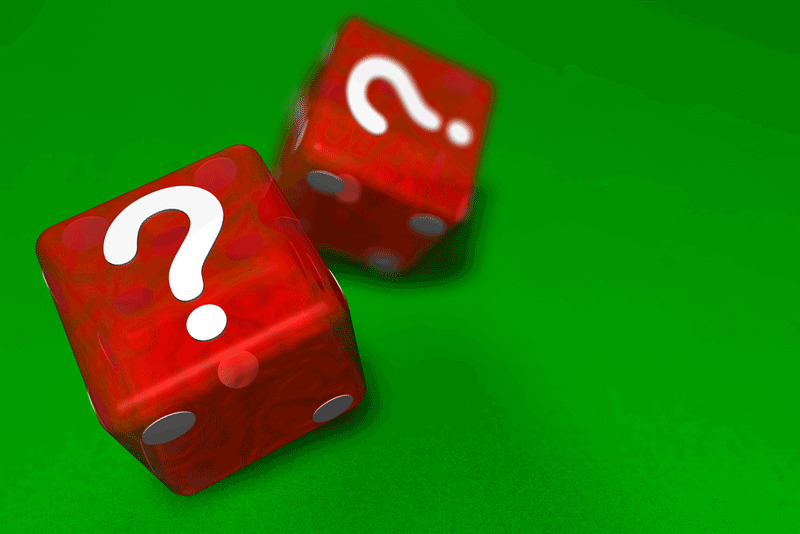
The so-called Nil Coalescing Operator is an interesting operator, that you can use for working with optionals.
Hint: This post has been updated to Swift 3
An Example
So imagine the following situation: You have an optional value anOptionalInt and you want to assign the value to a non-optional value. If the optional is nil, you want to assign a default value. You can solve this problem as follows:
var anOptionalInt: Int? = 10 var anInt: Int = 0 if anOptionalInt != nil { anInt = anOptionalInt! }
On the one hand this code is very long for the task, but it’s also very clear what’s going on. However, there are shorter solutions.
Ternary Conditional Operator
As in C, Swift has a so-called ternary conditional operator. There are a lot of discussions whether it’s a good idea or a bad idea to use it. I think in most cases it’s not a good idea because the code becomes very difficult to read. Let’s take a look at using the ternary conditional operator in our example:
var anOptionalInt: Int? = 10 var anotherOptional = (anOptionalInt != nil ? anOptionalInt! : 0)
This is of course much shorter. However, I have to read it at least twice to understand what’s going on. So I wouldn’t choose this approach.
Nil Coalescing Operator
For our example, there is a designated operator in Swift, the so-called nil coalescing operator. Let’s see how it works:
var anOptionalInt: Int? = 10 var anotherOptional = anOptionalInt ?? 0
The code is both very short and easy to read. When I’ve first heard about this operator, I wasn’t sure whether it’s a good idea to use it or not because I don’t like this kind of operators. But in this case I think this operator if definitely very useful, so I will give it a try.
What do you think? Is it a good idea to use the nil coalescing operator? Please provide your comment down below!
Resources
Title Image: @ TheUmf / shutterstock.com