Singletons in Swift
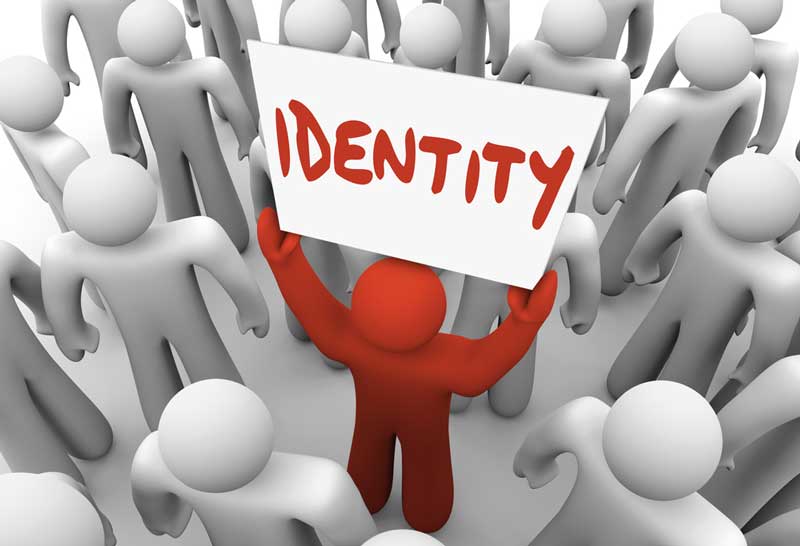
Some people love it, some people hate it – but in the end everybody uses it: the singleton pattern. It is used if just one instance of an object is desired. For example, that could be a database connection.
You can design a singleton in Swift as follows:
import Foundation private let _sharedInstance = SingletonObject() class SingletonObject { private init() { } class var sharedInstance: SingletonObject { return _sharedInstance } }
The init methode is private, so it is not possible to call it from outside of the source file. The only way to get access to an instance is by calling the sharedInstance property, which returns the global – but private – constant _sharedInstance .
This way, you get access to the singleton instance with
SingletonObject.sharedInstance
It is also possible to access the singleton directly through the globale constant if you remove the private keyword:
import Foundation let singletonObjectSharedInstance = SingletonObject() class SingletonObject { private init() { } }
This is a little bit shorter, but then you access the instance with just
singletonObjectSharedInstance
which is in my opinion bad practice.
Edit: A comment by Andrew has drawn my attention to another good solution. Since Swift 1.2 it is possible do declare static class properties. So you can implement the singleton like this:
final class SingletonObject { static let sharedInstance = SingletonObject() private init() { } }
Note: I made no statements about the thread-safety of these solutions. This will be discussed in another blog post.
References:
Image: @ iQoncept / shutterstock.com