Swift 2: guard
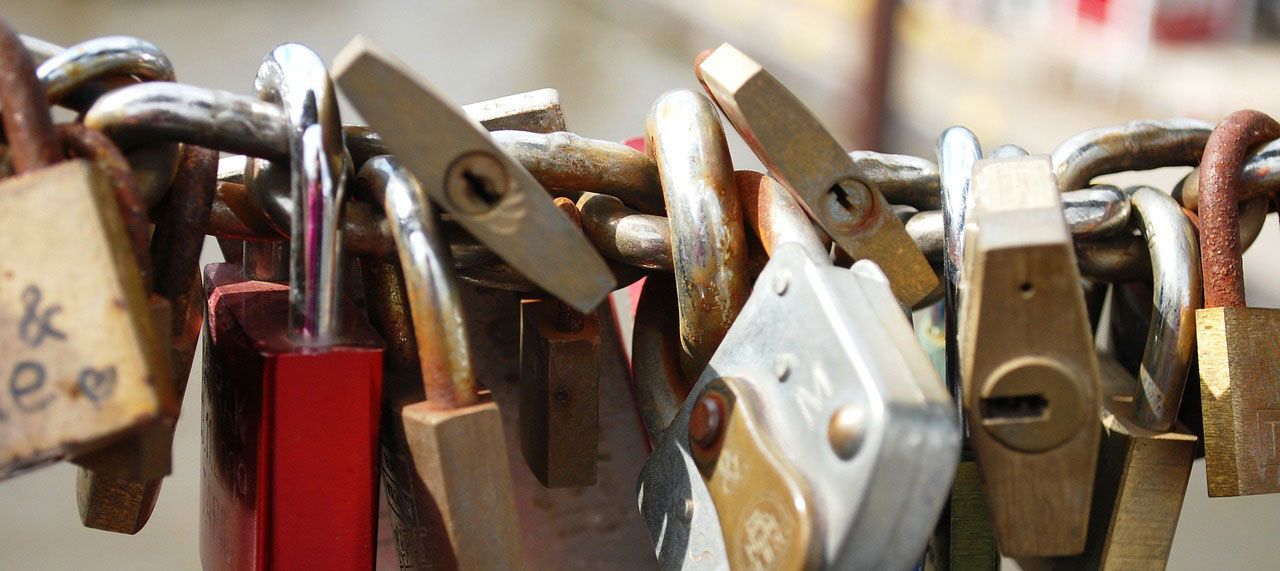
In Swift 2, there is a new keyword for handling the control-flow of a block of code. It guarantees, that a specific condition holds true for the further code. Otherwise, it ensures that the following code will not be executed.
Let us start with a simple example. Imagine you have a function, that has an optional as an argument:
func testItOld(a: Int?) { if let a = a { print("a: (a)") } else { print("not ok") } }
Because a is an optional, you need to check whether a is nil or not.
In this situation, guard gives you a simpler possibility:
func testItWithGuard(a: Int?) { guard let a = a else { print("not ok") return } print("a: (a)") }
In the guard condition, there is conditional binding of a . If it fails, the else part of the guard statement must ensure that the following code will not be executed. In this case, the function is returned.
After the guard statement, a is not an optional anymore, which makes the handling of optionals easier then before.
Generally, the guard statement can be used everywhere, where one of the following statements can be called:
return, break, continue, throw
If none of these keywords are called, there will be a compiler error!
So you can for example ensure, that a condition holds for a loop iteration:
var numbers: [Int?] = [1,2,3,nil,5] for a in numbers { guard let a = a else { continue } print("value (a)") }
Another good application case is throwing an error. Our previous example can be rewritten to
enum anErrorType: ErrorType { case numberNotValid } func testItWithGuard(a: Int?) throws { guard let a = a else { print("not ok") throw anErrorType.numberNotValid } print("a: (a)") }