Swift 2.0: defer
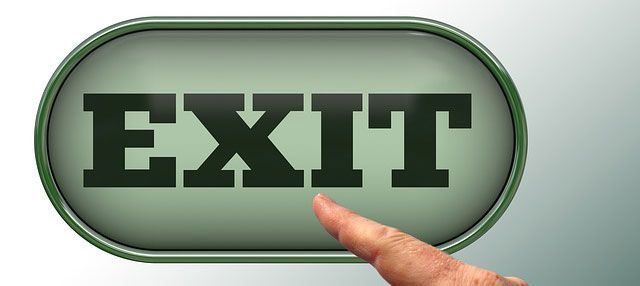
defer is another new keyword in Swift. With this statement you can declare clean-up code, that is executed just before the current scope ends. For example, this scope can be a function or a loop.
Let’s take a look at an example:
func aTestFunction(a:Int) { //lots of code... if a > 5 { print("1") print("clean-up") return } //lots of code... print("2") print("clean-up") }
If you want to run some clean-up code just before the function returns, you would insert them at two positions. And this is just a simple example.
But with defer you need to declare the clean-up code only once:
func aTestFunction(a:Int) { defer { print("clean-up") } //lots of code... if a > 5 { print("1") return } //lots of code... print("2") }
If you call this function with an argument of 3, the output will be
2 clean-up
You can place the defer statement at any position of the current scope, but it is considered as good practice to place it at the beginning of the current scope. It is also possible to have more than one defer block in the current scope. They are executed then in reverse order.
The defer block is also executed if the current scope ends due to a thrown error:
enum SomeErrorType: ErrorType { case anError } func aTestFunction(a:Int) throws { defer { print("clean-up") } //lots of code... if a > 5 { print("1") throw SomeErrorType.anError } //lots of code... print("2") }
Conclusion
With defer you can declare clean-up code that is executed if the current scope ends. This improves the readability of your code and the code quality overall.