Swift: map and flatMap
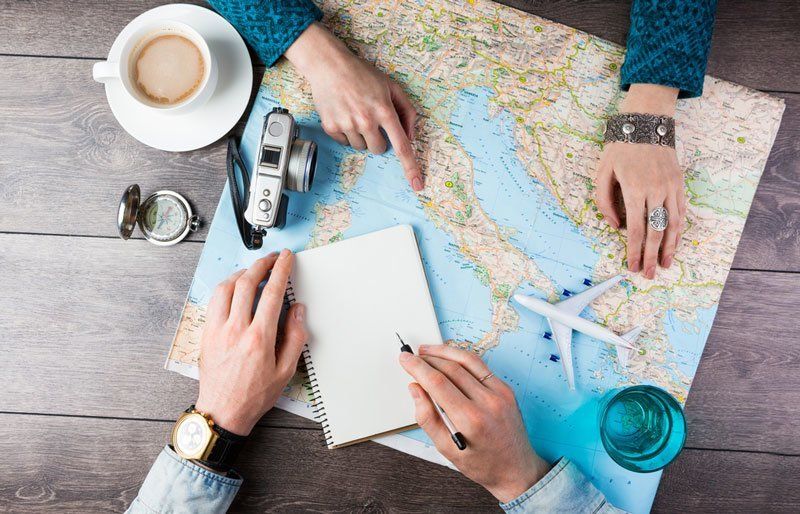
With the functions map and flatMap you can easily transform an array into a new one.
Hint: This post has been updated to Swift 3
Map
The function map can be called on an array and takes a closure that is called on each element of the array. This closure returns a transformed element and all these transformed elements build the new array.
This sounds complicated, but it is very straightforward. Imagine you have an array of strings:
let testArray = ["test1","test1234","","test56"]
The closure of the function map takes a string as a parameter because we call the function on an array of strings. In this example we want to return an array of integers that contains the length of the string elements. The return type of the closure is Int?.
let anotherArray = testArray.map { (string:String) -> Int? in let length = string.characters.count guard length > 0 else { return nil } return string.characters.count } print(anotherArray) //[Optional(5), Optional(8), nil, Optional(6)]
FlatMap
flatMap is like map, but it drops the elements that are equal to nil:
let anotherArray2 = testArray.flatMap { (string:String) -> Int? in let length = string.characters.count guard length > 0 else { return nil } return string.characters.count } print(anotherArray2) //[5, 8, 6]
Another difference to map is that optionals are converted to non-optionals, if they are not equal to nil.
References
Image: @ Fly_dragonfly / shutterstock.com