Swift Playground: The Monty-Hall-Problem
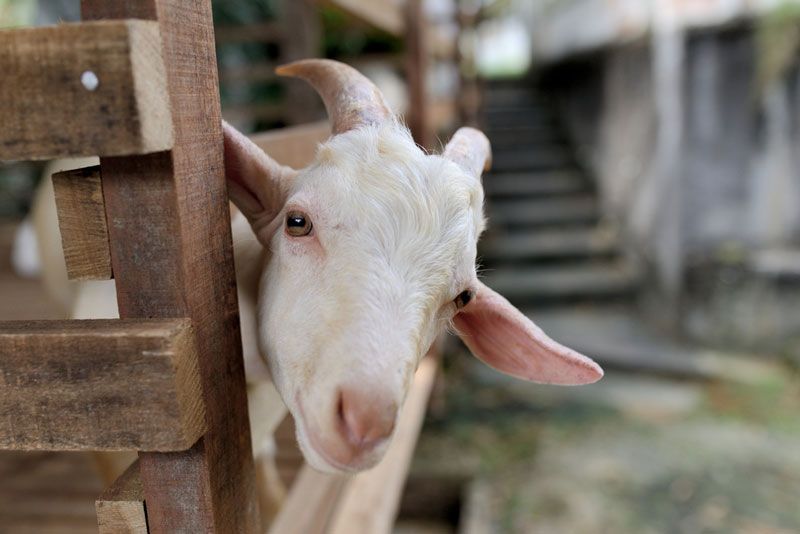
The Monty-Hall-Problem is a very confusing probability problem. Instead of doing mathematics, we will experiment on a Swift playground.
Hint: This post has been updated to Swift 3
The Monty-Hall-Problem
The idea is very simple. It is explained in Parade magazine in 1990:
Suppose you’re on a game show, and you’re given the choice of three doors: Behind one door is a car; behind the others, goats. You pick a door, say No. 1, and the host, who knows what’s behind the doors, opens another door, say No. 3, which has a goat. He then says to you, “Do you want to pick door No. 2?” Is it to your advantage to switch your choice?
In fact, it is to your advance to switch! If you switch, you have a probability of 2/3 to win the car. On the other hand, if you don’t switch, your probability is just 1/3. Of course you can proof this mathematically. But we want to experiment.
The Algorithm
The algorithm is very straightforward. First, you choose a door. Then, the host has to open one door, which has a goat. If your first pick is the car, he has to open one of the two other doors at random. If your first pick is a goat, he has to open the one remaining door, which has a goat. Finally, depending on your strategy, you switch or not.
The Playground
Let’s program a small playground. All together we have 10,000 rounds per strategy:
import UIKit enum Strategy { case Change case Stay } func play(strategy:Strategy,repeats:Int) -> Int { var wins = 0 for _ in 0..<repeats { let car = Int(arc4random_uniform(3)) var playerChoice = Int(arc4random_uniform(3)) if strategy == Strategy.Change { if playerChoice == car { var remainingDoors = [0,1,2] remainingDoors.remove(at: playerChoice) playerChoice = remainingDoors[Int(arc4random_uniform(2))] } else { playerChoice = car } } if car == playerChoice { wins += 1 } } return wins } var repeats = 100000 var winsStrategyChange = play(strategy: .Change, repeats: repeats) var winsStrategyStay = play(strategy: .Stay, repeats: repeats) var quoteStrategyChange = Double(winsStrategyChange) / Double(repeats) var quoteStrategyStay = Double(winsStrategyStay) / Double(repeats)
The Results
Of course the results are changing a little bit every time you are running the playground, but are always very similar. For example, one result was:
- winsStrategyChange: 66,461
- winsStrategyStay: 33,509
- quoteStrategyChange 0,66461
- quoteStrategyStay: 0,33509
The result are as the theory predicts. If you don’t switch the door, you are wining in about 33 percent of the cases. On the other hand, if you do switch the door, you are winning in about 66 percent of the cases.
[thrive_text_block color=”blue” headline=”Conclusion”]It makes a lot of fun to experiment in playgrounds. In this case, we were able to verify a theory that is a bit confusing at the first sight.[/thrive_text_block]
References
Image: @ Lim ChewHow / shutterstock.com
Wikipedia: Monty Hall Problem