The A-Z Of iOS Development
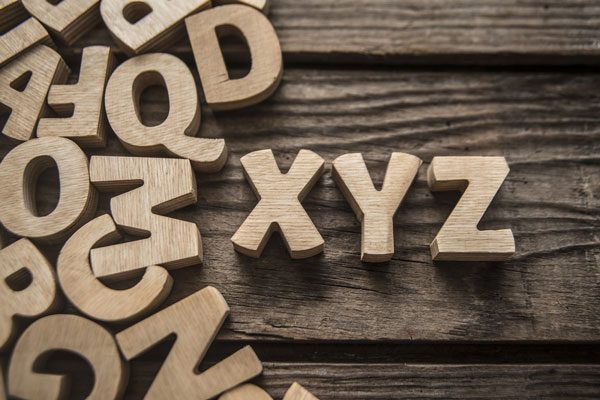
The A-Z of iOS development – or at least a part of it…
Autolayout
Autolayout is one of the key technologies for defining the layout of iOS apps. At the early days of iOS development, there were just very few screen sizes, so you were able to define the layout very easily. But now there are a lot of different devices and screen sizes, so you have to take a more general approach. With Autolayout you define some attributes like spacing between elements and the layout system will take care of everything else. It works very good, even though it’s not easy to understand at the beginning. A good introduction to this topic is this WWDC 2016 video: Making Apps Adaptive, Part 1.
Best Practices
In iOS development there are a lot of best practices for code style, software architecture, design, layout and many other areas. The bad news is that there is a lot to learn. It’s also a lot of fun though! And the learning process will never end…
CoreData
When it comes to data persistence, Core Data is the standard way to go on iOS. Of course there are other ways to persist data as well and all of these ways have their use cases. However, in most cases it does make sense to use CoreData. It’s fast, it’s reliable and after a little bit of learning it’s not even so difficult to use 😉
Developer Portal
The Apple developer portal is the central source for iOS development. Here you can access your developer account, upload apps to the iOS app store, access the developer documentation, download new beta versions of Xcode, iOS and MacOS and much more.
Error Handling
This is a topic many developers don’t like. But let’s face it: There will be errors when an app is running. This error could be for example a not responding server. In order to provide a good user experience, it’s your job to prepare the app for these situations and to respond appropriately.
Foundation
Foundation is one of the major frameworks you are using in iOS development. It’s not iOS specific, so the same framework can be used in MacOS development or even for server side development with Swift. The framework includes classes for different functionalities: Data storage, dates and times, application coordination and timing, object distribution and persistence, URL loading and many more. For more details take a look at the Foundation documentation.
Git
When you are developing an iOS app, you should always use a Git repository. It helps you to organise the progress of your work and you can work together with other developers on the same project. You can also use a Git repository in the cloud, so that your work is also backed up automatically. Good cloud services for Git are GitHub and BitBucket.
Hello World!
If you are very new to programming, this could probably be the name of your very first application.
iCloud
Since we are using a lot of different devices, it’s important to synchronise the data between all these devices. For Apple devices, iCloud is the way to go.
JSON
Very often you will use so-called web services. For example, if you want to write a Twitter client, you can use Twitter’s web services to request the user’s timeline and post new Tweets. Of course the data that’s exchanged between client and server needs to be structured in some way. Very often the JSON format is used for that.
Keyboard Shortcuts
If you are working the whole day with Xcode, you will find out very quickly that there are a lot shortcuts that will make your workflow much quicker. You should really invest some time to learn these shortcuts. Take a look a this post for more details about this topic: The 10 Best Xcode Shortcuts.
Learning
Software development is both difficult and fast changing. So one of the most important skills for software developers is the willingness to learn on a regular basis. There are several ways to stay up-to-date on iOS development though, so that you can pick your favourite way of learning.
Memory Management
Compared to desktop devices, iPhones and iPads don’t have a lot of memory. And iOS is very strict in terminating applications that are using to much memory. And of course no user likes it when an app crashes. So it’s your responsibility to ensure that the app does a good memory management jobs. There are some generell principles you should follow.
Never give up!
Well, that’s one of the most important things in iOS development. You will face days when you think that’s you should give up because nothing is working and it’s just too difficult. But then don’t give up! It’s very normal that we are facing those days and it’s our task then to persevere.
Objective-C
Let’s face it: Swift is the present and the future of iOS development. However, there are still a lot of projects that has been written in Objective-C and that needs to be maintained. In most cases it doesn’t make sense to convert a project from Objective-C to Swift, so it’s still important to have some Objective-C knowledge. However, learning Swift should be your priority.
Provisioning Profiles
Every app needs to be signed. For that, you need both a so-called certificate and a provisioning profile. The certificate is all about the developer. This way iOS can identify whether the owner of the developer account has created the app. The provisioning profile on the other hand is all about the app. For example, it specifies whether the app is builded for the App Store or for testing purposes. This sounds very simply, but you will encounter some problems with this topic as an iOS developer. Be prepared.
QuartzCore
Animations are very important in an iOS app and there are several ways to implement animations. The Core Animation classes from the QuartzCore framework is one of these ways.
Retain Cycles
As I’ve mentioned before, memory management is even in these days an important task. And there’s one thing that you should prevent at all costs: retain cycles. They occur when two objects have strong references to each other. Then, these objects can’t be deallocated and will always remain in the memory until the app uses to much memory and the operating systems decides to kill the app. I’ve written two posts about this topic: A Trick To Discover Retain Cycles and Retain Cycles, Weak and Unowned in Swift.
Swift
Swift is THE programming language for developing iOS applications. Of course there are still a lot of apps that has been written in Objective-C, but in my opinion you should start new projects always in Swift. And – of course – as a new iOS developer you should concentrate to learn Swift instead of Objective-C.
Testing
Testing is important. That holds true for every kind of software development, but in mobile development it’s particularly important because mobile is different: On the on hand you have an unstable internet connection and the performance of the devices is bad compared to normal computer. But on the other hand the user has high expectations for the user experience.
UIKit
Besides Foundation, UIKit is the most important framework in iOS development. It provides you all the user interface elements you need. And even every control you are developing on your own is based on an element that comes from UIKit.
View Controller
UIViewController is one of the most important user interface classes. Every screen has a view controller and it’s its job to coordinate the so-called model and the view.
WWDC
The WWDC is Apple’s annual developer conference. It always takes place in San Francisco. On the WWDC new technology gets announced and there’s always a lot to learn. If you don’t have the opportunity to visit the WWDC personally, you can access all session videos on Apple’s developer portal.
Xcode
Xcode is the IDE for developing iOS, MacOS, watchOS and tvOS applications. You can download it either from the App Store or from the Apple developer portal.
You
The most important part in developing an app is you. You are the developer and it’s up to you to learn all the necessary skills, have the right ideas and having the persistence to developer an app. Good luck!
Zombies
Yes, that’s right: There are zombies in iOS development… Trust me: You will have an encounter!
References
Image: @ maradon 333 / shutterstock.com