Xcode: Navigating Through Your Project
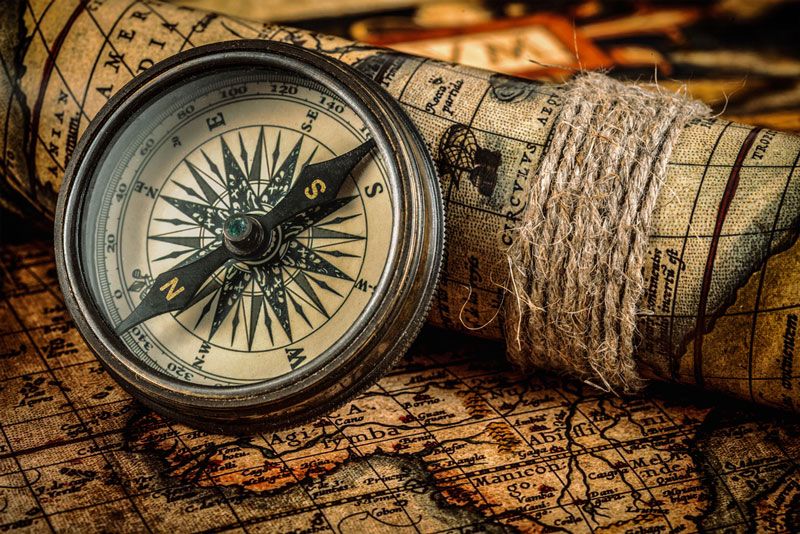
If you are an iOS developer, you are navigating through your project the whole day. You can save a lot of time and increase your productivity if you know some tricks that make your life easier.
Landmarks
In Swift landmarks are the successor of Objective-C’s pragma marks. Using them you get a good navigation help in the jump bar.
There are three types of landmarks:
- MARK:
- FIXME:
- TODO:
You can insert them as comments into your code:
import UIKit class ViewController: UIViewController { //MARK: - Properties lazy var anArray = ["String1","String2"] //MARK: - UIViewController override func viewDidLoad() { super.viewDidLoad() //TODO: Localize labels } override func didReceiveMemoryWarning() { super.didReceiveMemoryWarning() //TODO: Implement memory warning handling } //MARK: - UserAction @IBAction func buttonPressed(sender: AnyObject) { //FIXME: Crash at runtime println("\(anArray[2])") } }
Then, by clicking on the jump bar (the bar above the source code editor) you get additional hints:
If a class has a lot of lines, this way you get very quickly an overview and you can for example directly jump to the part of the class where a certain protocol is implemented. Furthermore, you are more careful at placing methods at the right position in the code.
Search
There are three important search types:
- Search in the current file
- Global search in the project
- Filename search
You can search in the current file if you click on “Find -> Find…” or by using the keyboard shortcut Command + F. Then a search bar appears and you can enter a search string.
The global search can be accessed through the “Find navigator” in the “Navigator” on the left side. After entering a search string all search results from the whole project will be presented.
The keyboard shortcut for the global search is Shift + Command + F.
One of the most useful features in Xcode is the Filename search. You can open it with the keyboard shortcut Shift + Command + O. A search box appears in the middle of the screen. After you have entered a search string a list appears with all files whose filenames contain the search string.
Groups
It is a very good advise to structure your project in a clean and consistent way. There are several ways how you can do this. However, there is on way that is very useful: You create three groups “Model”, “View” and “Controller”. Then you can sort in your source code files according to the MVC belonging. This way you are always reminded to think about your app architecture. For more information about the MVC pattern take a look at my “The MVC-Trap” post.
Callers
If the cursor is on a method name in the source code editor you can click in the left corner of the jump bar. Then you can click on “Callers” and a list with all calls of this function in the whole project is revealed.
[thrive_text_block color=”blue” headline=”Conclusion”]It is very advisable to take some time and learn some ways to navigate through a project and learn some keyboard shortcuts. This can save you a lot of time in your daily working routine.[/thrive_text_block]
References:
The 10 Best Xcode Shortcuts
The MVC-Trap
Image: @ f9photos / Shutterstock.com